Introduction to Multithreading
A program can be divided into a number of small processes. Each small process can be addressed as a single thread (a lightweight process). Multithreaded programs contain two or more threads that can run concurrently. This means that a single program can perform two or more tasks simultaneously. For example, one thread is writing content on a file at the same time another thread is performing spelling check.
In Java, the word thread means two different things.
- An instance of Thread class.
- or, A thread of execution.
An instance of Thread class is just an object, like any other object in java. But a thread of execution means an individual "lightweight" process that has its own call stack. In java each thread has its own call stack.
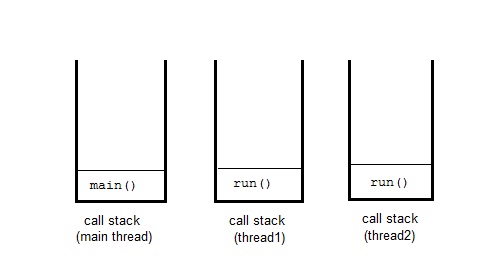
The main thread
Even if you don't create any thread in your program, a thread called main thread is still created. Although the main thread is automatically created, you can control it by obtaining a reference to it by calling currentThread() method.
Two important things to know about main thread are,
- It is the thread from which other threads will be produced.
- main thread must be always the last thread to finish execution.
class MainThread { public static void main(String[] args) { Thread t=Thread.currentThread(); t.setName("MainThread"); System.out.println("Name of thread is "+t); } }
Output :
Name of thread is Thread[MainThread,5,main]
Life cycle of a Thread
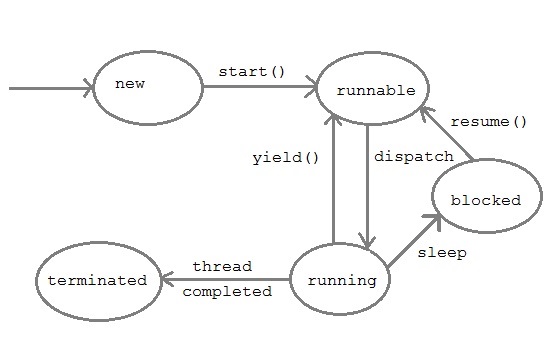
- New : A thread begins its life cycle in the new state. It remains in this state until the start() method is called on it.
- Runable : After invocation of start() method on new thread, the thread becomes runable.
- Running : A method is in running thread if the thread scheduler has selected it.
- Waiting : A thread is waiting for another thread to perform a task. In this stage the thread is still alive.
- Terminated : A thread enter the terminated state when it complete its task.
Thread Priorities
Every thread has a priority that helps the operating system determine the order in which threads are scheduled for execution. In java thread priority ranges between,
- MIN-PRIORITY (a constant of 1)
- MAX-PRIORITY (a constant of 10)
By default every thread is given a NORM-PRIORITY(5). The main thread always have NORM-PRIORITY.
No comments:
Post a Comment